Tags: pandas excel python pandas excel pandas csv python pandas csv pandas excel tutorial pandas csv tutorial pandas read_csv pandas to_csv pd read_csv pandas write csv python read excel python csv tutorial python write csv python excel pandas write pandas read excel pandas write excel pandas read csv how to read csv file in python read csv file in python csv python pandas read csv file how to read csv file in pandas read csv file in pandas
This tutorial covers how to read/write excel and csv files in pandas. We will cover,
1) Different options on cleaning up messy data while reading csv/excel files
2) Use convertors to transform data read from excel file
3) Export only portion of dataframe to excel file
Topics that are covered in this Python Pandas Video:
0:00 Introduction
1:26 Read CSV file using read_csv() method
2:39 Skip rows in dataframe using "skiprows"
4:44 Import data from CSV file with "null header"
6:28 Read limited data from CSV file
7:19 Clean up messy data from file "not available" and "n.a." replace with "na_values"
9:01 Supply dictionary for replace with "na_values"
11:40 Write dataframe into "csv" file with "to_csv() method"
15:27 Read excel file using read_excel() method
18:03 Converters argument in read_excel() method
20:17 Write dataframe into "excel" file with "to_excel() method"
22:56 Use ExcelWritter() class
25:13 All properties for Read Write Excel CSV File
Do you want to learn technology from me? Check https://codebasics.io/?utm_source=description&utm_medium=yt&utm_campaign=description&utm_id=description for my affordable video courses.
Very Simple Explanation Of Neural Network: https://www.youtube.com/watch?v=ER2It2mIagI
Code (jupyter notebook link): https://github.com/codebasics/py/tree/master/pandas/4_read_write_to_excel
Next Video:
Python Pandas Tutorial 5: Handle Missing Data: fillna, dropna, interpolate: https://www.youtube.com/watch?v=EaGbS7eWSs0&list=PLeo1K3hjS3uuASpe-1LjfG5f14Bnozjwy&index=5
Popular Playlist:
Complete python course: https://www.youtube.com/playlist?list=PLeo1K3hjS3uv5U-Lmlnucd7gqF-3ehIh0
Data science course: https://www.youtube.com/playlist?list=PLeo1K3hjS3us_ELKYSj_Fth2tIEkdKXvV
Machine learning tutorials: https://www.youtube.com/playlist?list=PLeo1K3hjS3uvCeTYTeyfe0-rN5r8zn9rw
Pandas tutorials: https://www.youtube.com/playlist?list=PLeo1K3hjS3uuASpe-1LjfG5f14Bnozjwy
Git github tutorials: https://www.youtube.com/playlist?list=PLeo1K3hjS3usJuxZZUBdjAcilgfQHkRzW
Matplotlib course: https://www.youtube.com/playlist?list=PLeo1K3hjS3uu4Lr8_kro2AqaO6CFYgKOl
Data structures course: https://www.youtube.com/playlist?list=PLeo1K3hjS3uu_n_a__MI_KktGTLYopZ12
Data Science Project - Real Estate Price Prediction: https://www.youtube.com/watch?v=rdfbcdP75KI&list=PLeo1K3hjS3uu7clOTtwsp94PcHbzqpAdg
To download csv and code for all tutorials: go to https://github.com/codebasics/py, click on a green button to clone or download the entire repository and then go to relevant folder to get access to that specific file.
π My Website For Video Courses: https://codebasics.io/?utm_source=description&utm_medium=yt&utm_campaign=description&utm_id=description
Need help building software or data analytics and AI solutions? My company https://www.atliq.com/ can help. Click on the Contact button on that website.
#οΈβ£ Social Media #οΈβ£
π Discord: https://discord.gg/r42Kbuk
πΈ Dhaval's Personal Instagram: https://www.instagram.com/dhavalsays/
πΈ Codebasics Instagram: https://www.instagram.com/codebasicshub/
π Facebook: https://www.facebook.com/codebasicshub
π± Twitter: https://twitter.com/codebasicshub
π Linkedin (Personal): https://www.linkedin.com/in/dhavalsays/
π Linkedin (Codebasics): https://www.linkedin.com/company/codebasics/
π Patreon: https://www.patreon.com/codebasics?fan_landing=true

or
Question

Very helpfull video but i wish to see web scraping in your tutorial
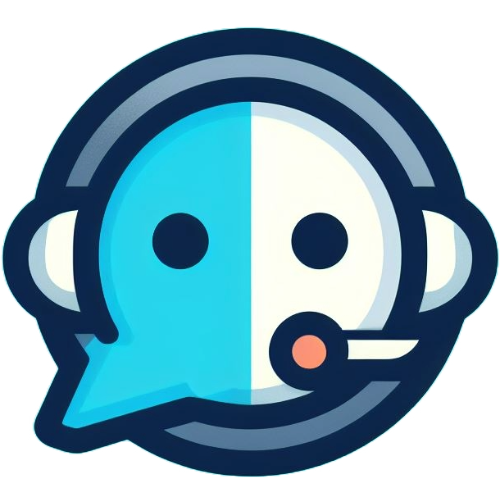

Hey can we add a new row with the functio used in Converters. Suppose we are reading Fuel Type Column which has Petrol, Diesel, CNG , xtra premium and then we want to add a new column say Fuel_price reading the values for each row of the column Fuel_Type, Please let me know if that's possible.
Any way I love the way you explain, specially for working professional like me who don't have time to study the document.
Thank you so much <3
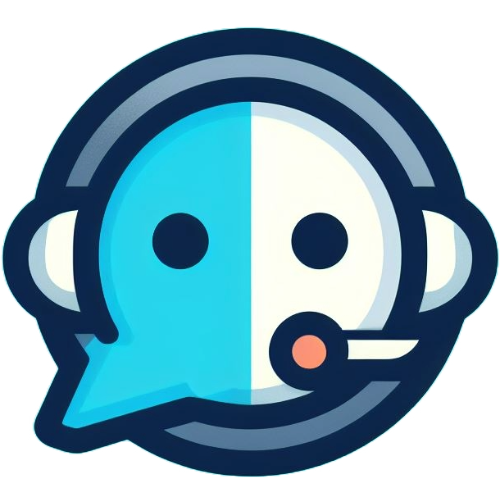

βThose who know, do. Those that understand, teach.β βAristotle
thank you very much sir for your effort .
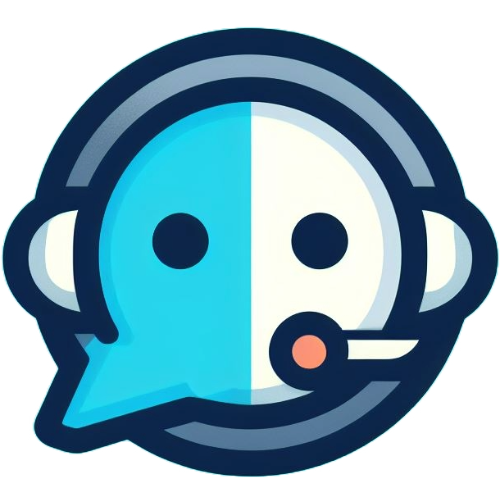

4:10 can I skip multiple rows?
Answer as soon as possible. Please.
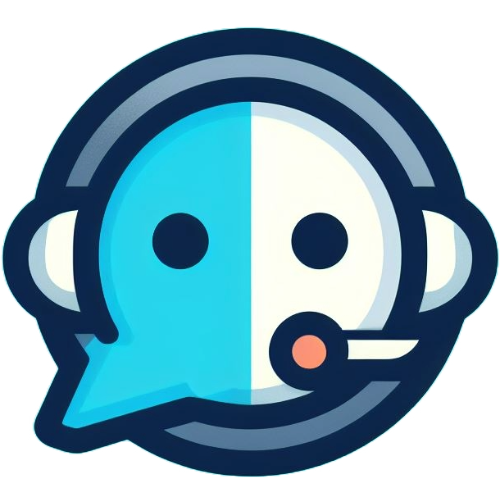

How can I add new column to the existing CSV file which is difference of values of two other cells in same CSV file?
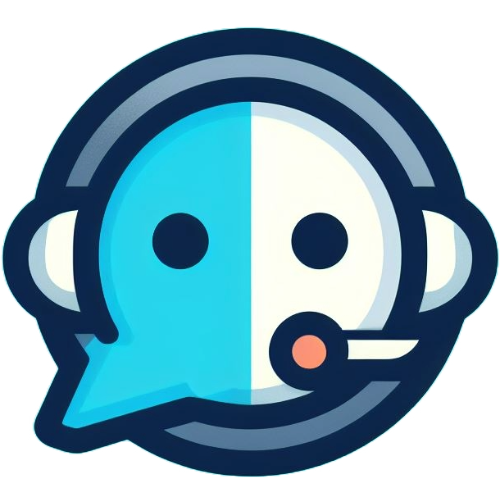

Seriously i never know that read function could be a bit challenging too
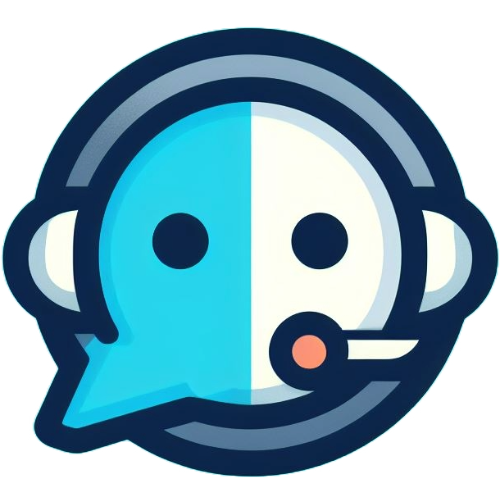