Tags: java java basics to advanced OOPs fundamentals inheritance in java polymorphism in java static polymorphsim dynamic polymorphism overloading overriding data abstraction in java data encapsulation in java java full course core java core java concepts shrayansh jain object oriented programming in depth java oops concept java oops java in hindi java basics
There are 4 pillars of OOPs:
- Inheritance,
- Polymorphism,
- Abstraction,
- Encapsulation.
i have all 4 in detailed with different examples. And interview questions which can be asked on top of it.
#java #objectorientedprogramming

or
Question

@sheyansh
In polymorphism, can we change access modifier and exception if any in case of overriding ?
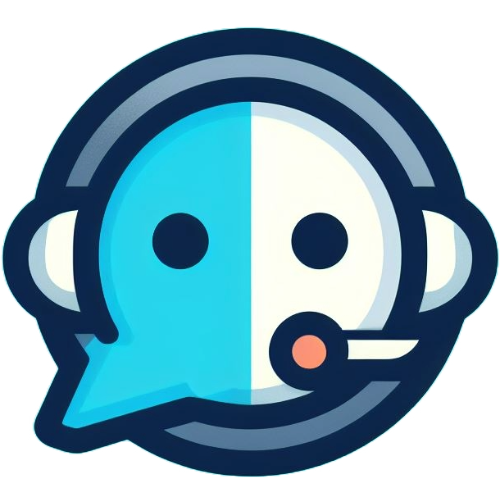

It is mentioned in Procedural or Functional programming that reusability is not possible but we can call the function again and reuse. Right ?
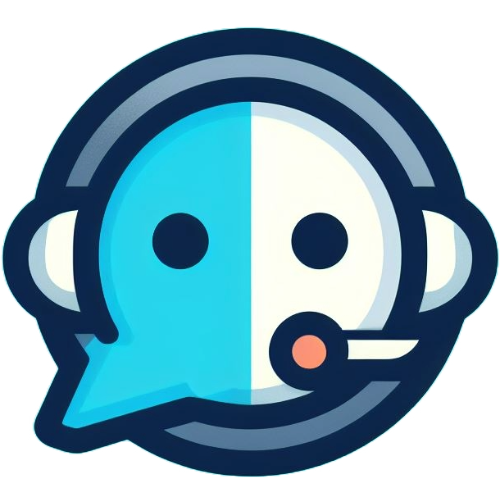

Pls correctly pronounce the word data, it's not datha it is daeta
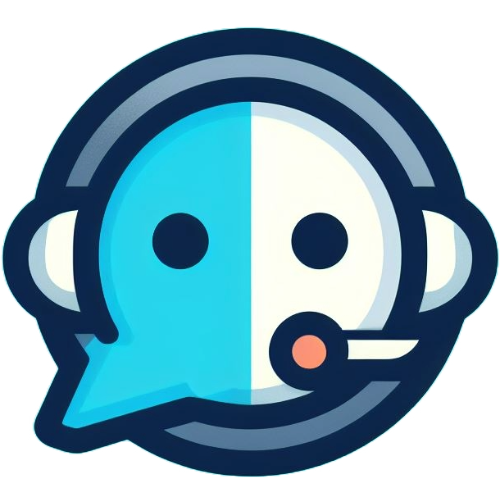

Class is a blueprint/template/skeleton/stencil/mold by which we can create objects.
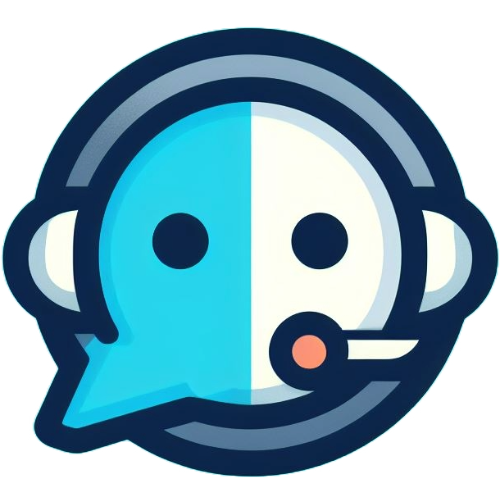

In polymorphism dynamic dispatch is missing. Anyways, great video simple & crisp.
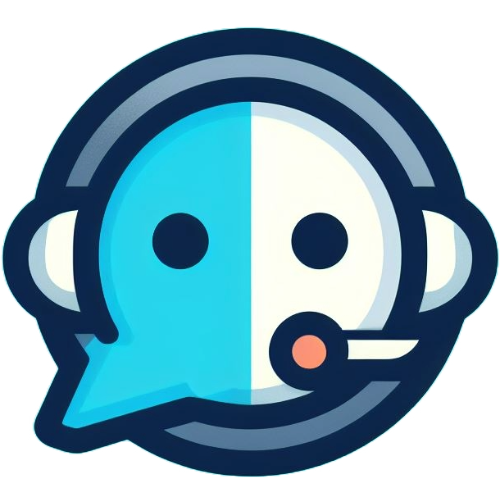

how to acheieve composition and aggregation in java . can you plz tell
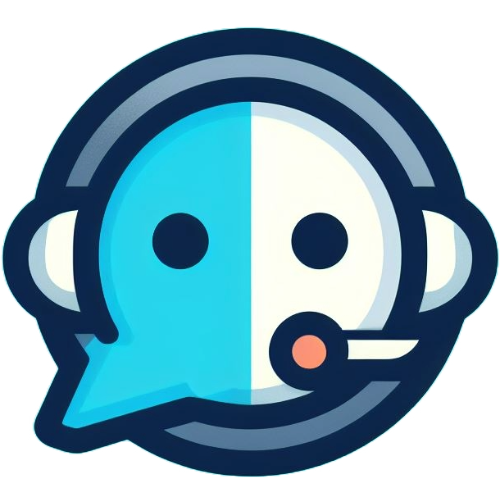

Awesome explanations...so simplified. Thanks a lot βΊοΈ
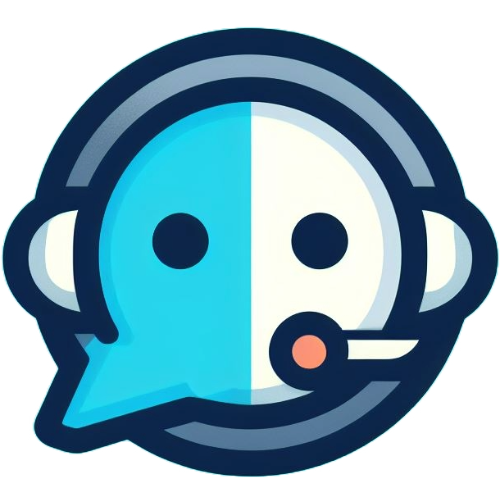

Hi shreyansh What is the use of private class if we can't access it. Can you tell any real-life example.
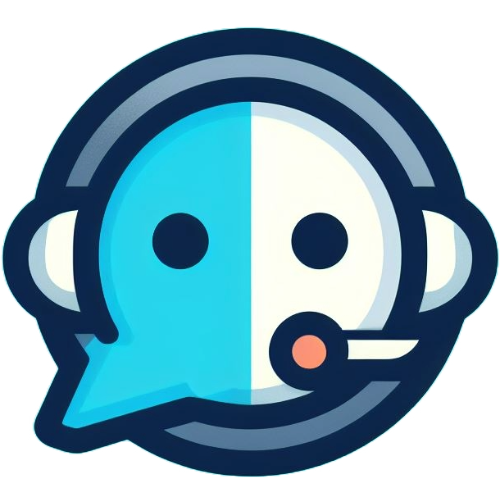