Tags: Java Recursion recursive java java recursive recursion java java recursive function recursion in java recursive function in java recursion Merge Sort Recursion (computer Science) Java (programming Language)
Get the Code: http://goo.gl/S8GBL
Welcome to my Java Recursion tutorial. In this video, I'm going to cover java recursion in 5 different ways. I figured if I show it using many different diagrams that it will make complete sense.
A recursive method is just a method that calls itself. As these calls are made the problem gets simpler until you reach a condition that leads to the method no longer making calls upon itself. This is known as the base case.

or
Question

Actually, for triangular numbers, you can calculate it like this:
public int getTriNum(int num) {
return (num + (num * (num - 1) / 2));
}
Or a bit more understandably:
public int getTriNum(int num) {
for(int i = 1; i <= num; i++) num += i;
return num;
}
And for factorials, you can also do it without recursion:
public int factorial(int num) {
for(int i = 1; i < num; i++) num *= i;
return num;
}
But factorial still is a pretty good example, nevertheless.
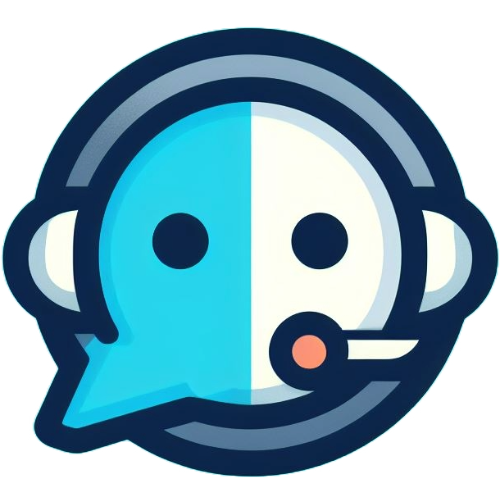

Why we need recursion if we have "while" or "if" ? Can you explain?
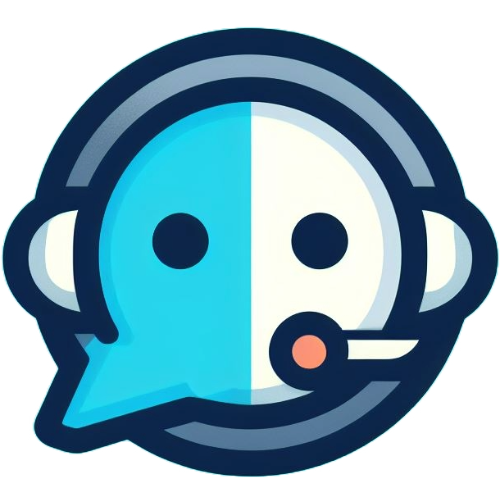

Could explain the difference between when one include the "static" key word to a variable/method versus when you don't? Thank u, great video thou :)
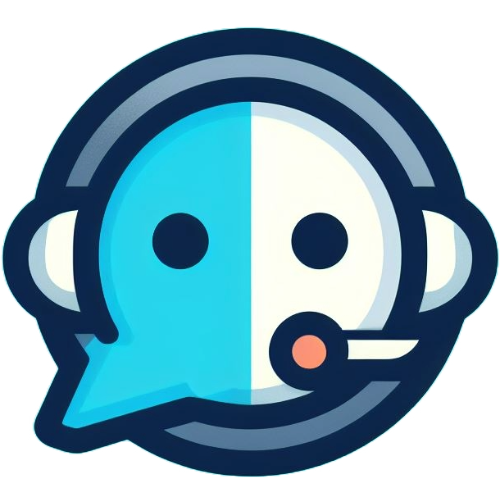

I don't mean to seem unappreciative, and though it seems like you know your stuff, you definitely go way too fast for a newb to absorb it. I feel like I barely picked anything up from it. Don't assume we know everything I have only had 8 weeks of Java before this and this is kind of abstract so it helps if you go a bit slower and perhaps explain things a bit more simply so we get it. I felt as if you whizzed through it so fast I might need to rewatch it 25 times before I really got what you were saying. The examples were good but it was like watching someone on super fast speed tell me how recursions work lol. Obviously, if you could if you slow down just a tad and rather than show many fast examples, show one or two slower explanations. Thanks for giving us the code to check out.
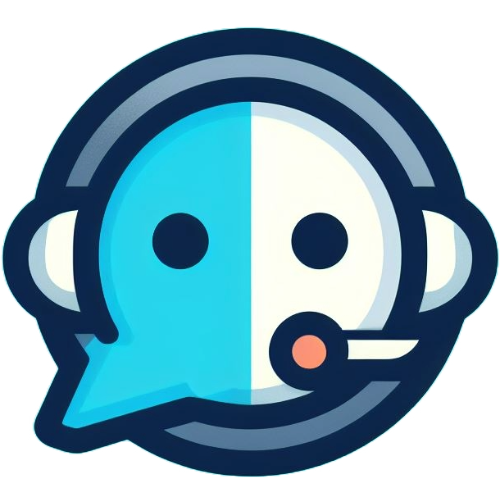