Tags: python data classes python data class python dataclass python data class tutorial dataclasses python 3.6 dataclasses python 3.7 data classes python data classes data class python storing data data-oriented class struct c# tutorial data class data vs behavior class python python tutorial learn python python 3.9 tutorial python 3.8 python 3.7 python 3.9.1 python 3.9 python 3.9.2 data classes python 3.7 data classes vs data
This video is a tutorial about Python's dataclasses. I take you through an example that shows what you can do with them. Python data classes are - as you'd expect - in particular suitable to model classes that represent data, and as such they offer easy mechanisms to initialize, print, order, sort, and compare data.
Note that although I'm using a sort_index attribute, strictly speaking that's not needed in this case, because a data class uses a tuple of its attributes in the class definition as the default for sorting. I'm not a fan of this kind of hidden behavior, so I prefer to do it explicitly (using something that is called sort_index in this case). Another advantage of using a separate field, is that you can do more complicated ordering, using for example a weighted combination of age and strength.
đĄHere's my FREE 7-step guide to help you consistently design great software: https://arjancodes.com/designguide.
đ Courses:
The Software Designer Mindset: https://www.arjancodes.com/mindset
The Software Designer Mindset Team Packages: https://www.arjancodes.com/sas
The Software Architect Mindset: Pre-register now! https://www.arjancodes.com/architect
Next Level Python: Become a Python Expert: https://www.arjancodes.com/next-level-python
The 30-Day Design Challenge: https://www.arjancodes.com/30ddc
đ GEAR & RECOMMENDED BOOKS: https://kit.co/arjancodes.
Some interesting links:
- https://realpython.com/python-data-classes/
- Frozen instances: https://docs.python.org/3/library/dataclasses.html#frozen-instances
- If you want to go 'next-level' with dataclasses, check out Pydantic (https://pydantic-docs.helpmanual.io/). Pydantic enforces type hints at runtime, and offers a really nice extension for data validation.
- The code from this video is available here: https://github.com/ArjanCodes/2021-dataclasses
đŹ Join my Discord server here: https://discord.arjan.codes
đŠTwitter: https://twitter.com/arjancodes
đLinkedIn: https://www.linkedin.com/company/arjancodes
đ”Facebook: https://www.facebook.com/arjancodes
đ Chapters:
0:00 Intro
0:33 Behavior-driven vs data-driven classes
2:11 Explaining the example
3:31 Creating a dataclass
4:37 Sorting and comparing
6:58 Default values
7:40 Creating read-only (frozen) objects
8:55 String representation of data
9:34 Final thoughts
#arjancodes #softwaredesign #python
Thumbnail photo background by Markus Spiske: https://unsplash.com/@markusspiske
DISCLAIMER - The links in this description might be affiliate links. If you purchase a product or service through one of those links, I may receive a small commission. There is no additional charge to you. Thanks for supporting my channel so I can continue to provide you with free content each week!

or
Question

@ArjanCodes
I wanna ask a little.
Is it ok if I can do like this
@dataclass
class Test:
my_list = ["item1", "item2", "item3"]
or there's a better approach for that?
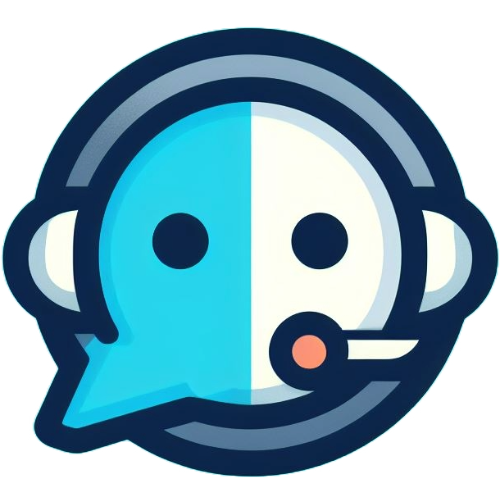

I stopped using data classes when I discovered pydantic
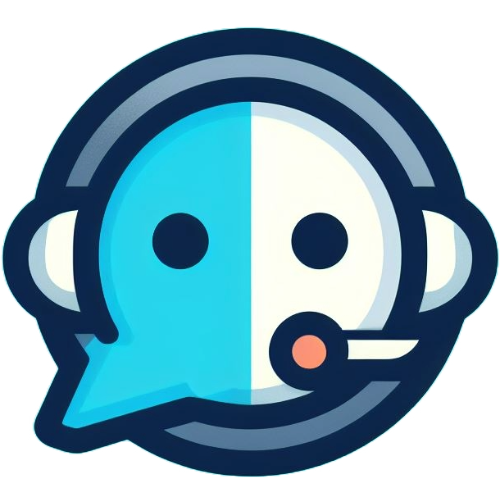

Thanks for the video but my question is what is the benefit of doing it that way vs turning that data into a DataFrame using Pandas for example? I'm just wondering if there are specific reasons/benefits due to some limitation or whatever I'm fairly new and still learning. Thanks in advance!
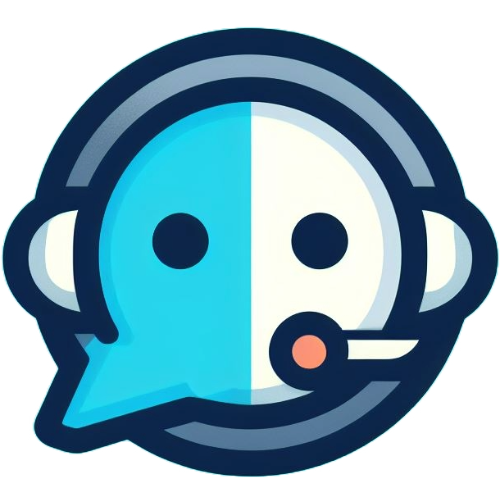

I really like this! It feels like modern python is much more robust and can be better self-documented and typed nowadays.
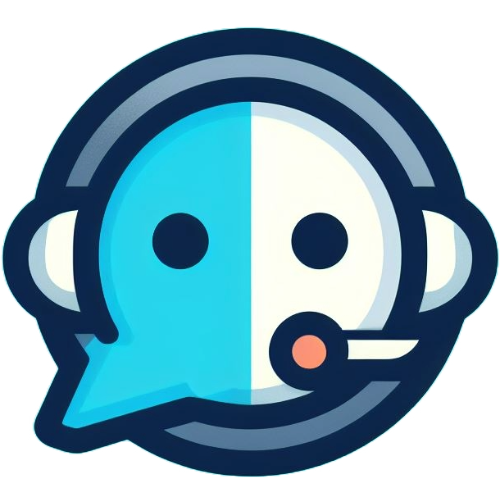

Awesome video, as always! I was going to ask about your thoughts on Pydantic, but then expanded the video description and noticed you were already way ahead of me. :D
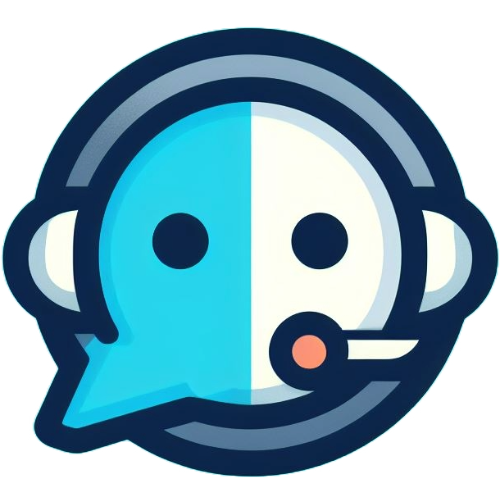

Thanks a lot for your explanation!
Exists some uses cases when it's better not to used dataclass and better use a regular class?
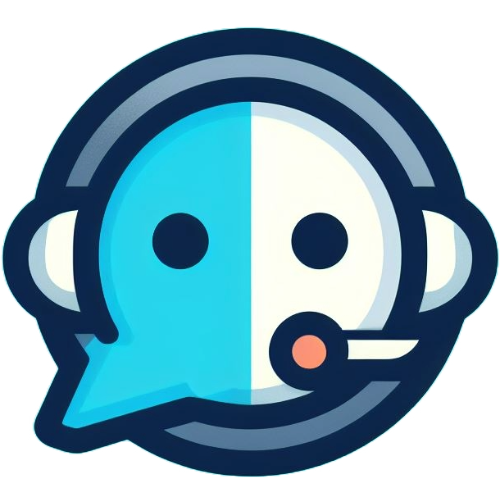

I've never been totally sold on data classes. To me, the functionality has been available forever in the form of dunder methods, @property decorators, and such. Am I being unreasonable here? Is there any sort of performance gain to data classes? Thanks for the excellent video as always!
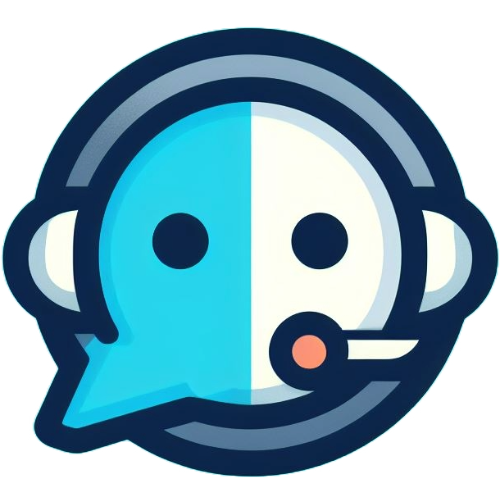

Great videos! I find your content really easy to follow and I always leave thinking of stuff I could try on my code. Like other people here, I often end up using mostly pandas and functions in my day to day code for data analysis tasks. Pandas is a lifesaver in so many of those. However, sometimes I end so deep in dataframes that juggling all of them, doing merges, etc, becomes a bit of a memory exercise. I feel dataclasses would probably come in handy in those situations, but it is hard to identify the points at which I should move between the two approaches. Input welcome!
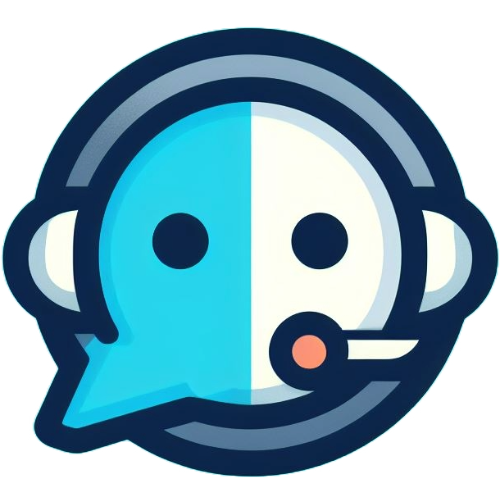

Is there an advantage to using the sort_index approach over dunder methods like lt, gt, etc?
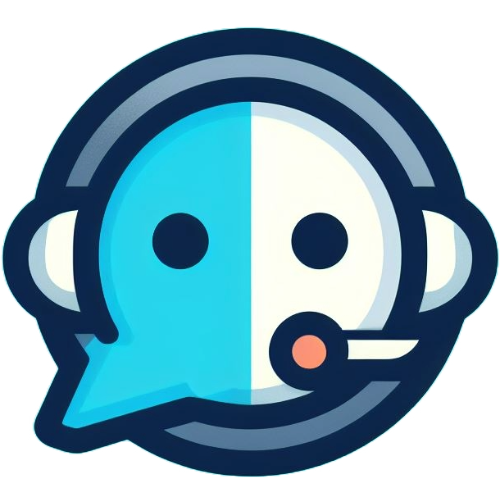

Great video, thanks!
Also, hi Reddit. :D
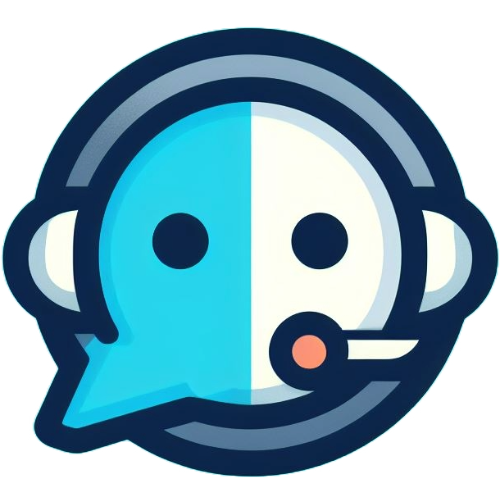