Tags: java codingwithjohn coding with john java beginner lesson junit junit testing in java junit testing in java intellij java unit testing java unit testing intellij unit testing unit testing intellij junit tutorial for beginners junit tutorial junit tutorial intellij java unit testing for beginners
Full tutorial on creating Unit Tests in Java with JUnit!
Do you write Java code that you're just not confident is 100% right? You can create unit tests using the JUnit testing framework to verify your Java code, and I'll show you exactly how to do it.
Many beginners don't know there's a great way to test their code with simple, fast tests and make sure their code is working exactly how they think it is.
The IntelliJ testing tools also make creating and running your Unit Tests super simple.
In this beginner's Java tutorial video, we'll do a full walkthrough of why and how you can create your own unit tests!
Learn or improve your Java by watching it being coded live!
Hi, I'm John! I'm a Lead Java Software Engineer and I've been in the programming industry for more than a decade. I love sharing what I've learned over the years in a way that's understandable for all levels of Java learners.
Let me know what else you'd like to see!
Links to any stuff in this description are affiliate links, so if you buy a product through those links I may earn a small commission.
📕 THE best book to learn Java, Effective Java by Joshua Bloch
https://amzn.to/36AfdUu
📕 One of my favorite programming books, Clean Code by Robert Martin
https://amzn.to/3GTPVhf
🎧 Or get the audio version of Clean Code for FREE here with an Audible free trial
http://www.audibletrial.com/johncleancode
🖥️Standing desk brand I use for recording (get a code for $30 off through this link!)
https://bit.ly/3QPNGko
📹Phone I use for recording:
https://amzn.to/3HepYJu
🎙️Microphone I use (classy, I know):
https://amzn.to/3AYGdbz
Donate with PayPal (Thank you so much!)
https://www.paypal.com/donate/?hosted_button_id=3VWCJJRHP4WL2
☕Complete Java course:
https://codingwithjohn.thinkific.com/courses/java-for-beginners
https://codingwithjohn.com

or
Question

The classes I want to do my unit test on, should they be in their own separate .java source code file? What if the custom class I want to test is written in a java source code file that also contains the main method, where should my unit test be written in that scenario? Great video!
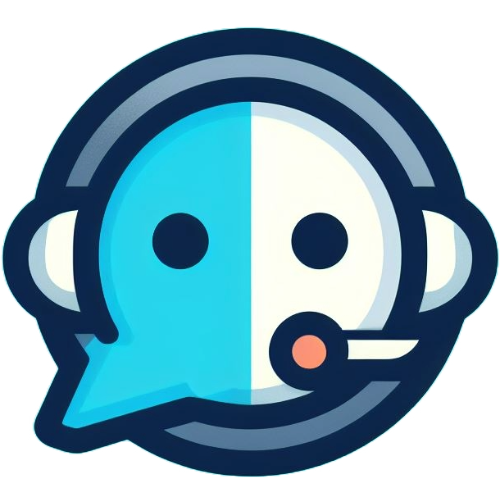

I heard that some people even write their unit tests first before writing their source code.
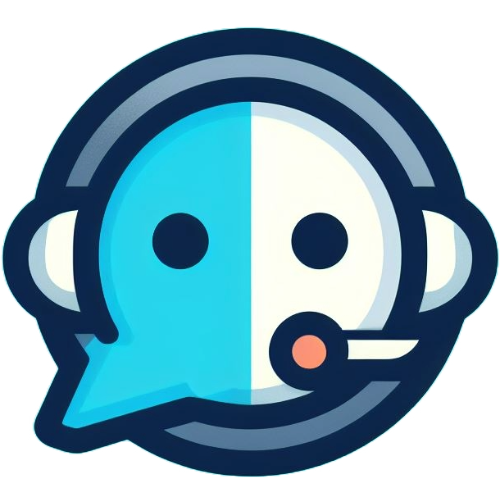

Had an assignmen to perform unit testing in Java usign the Junit . Was recommended to watch this video of yours . Your doing a great job ceating content for beginners like me Sir John . Had to subscribe ! Keep up the good and humble work !😇
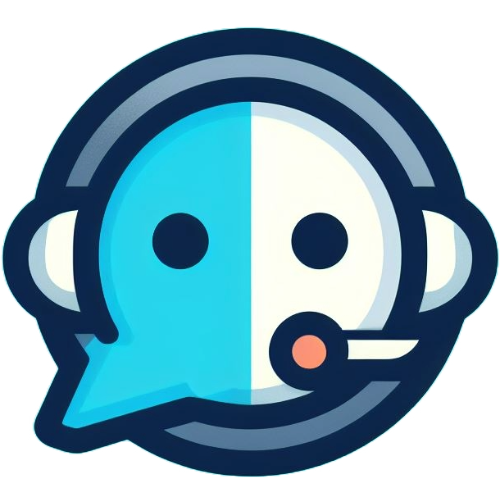

John, thank you for the great video on JUnit. My question is on code coverage, how it works? for example, if the condition is <=80, does it need 2 test cases (1 for =80 and one for <80) to make that line of code 100% covered? or does it just counts going through code once is considered as covered?
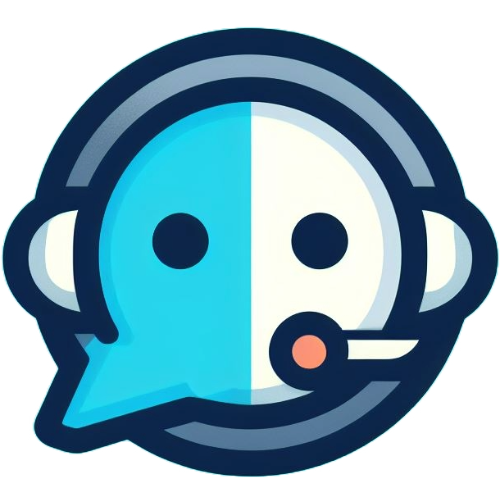

Can you help with this code....Here is the first part of the method. For some reason intelliJ does not recognize the method "determineLetterGrade" in my test. It doesn't compile nor show as a method option. Please advise!
public char determineLetterGrade(int numberGrade) {
if (numberGrade < 0) {
throw new IllegalArgumentException("grade must be 0 or higher");
}...
@Test
void determineGradeF() {
GraderTest grader = new GraderTest();
assertEquals(grader.determineLetterGrade(59));
}
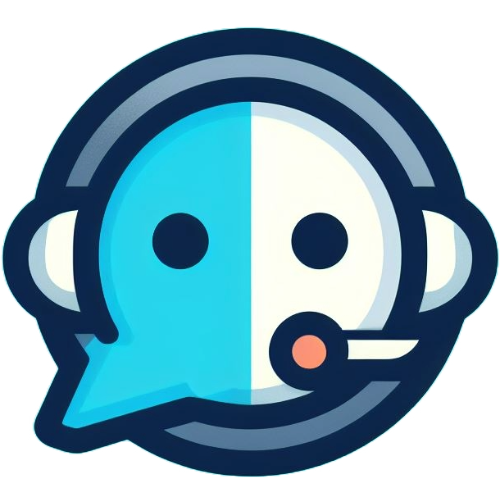

Hi, great video! It would be even better if you could cover Mockito, as it is usually used alongside JUnit.
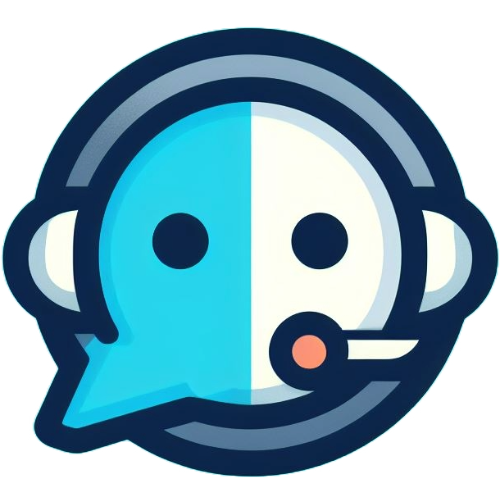

i dont understand why i would do this? seems alot more complicated than just checking if function(2,2)==4
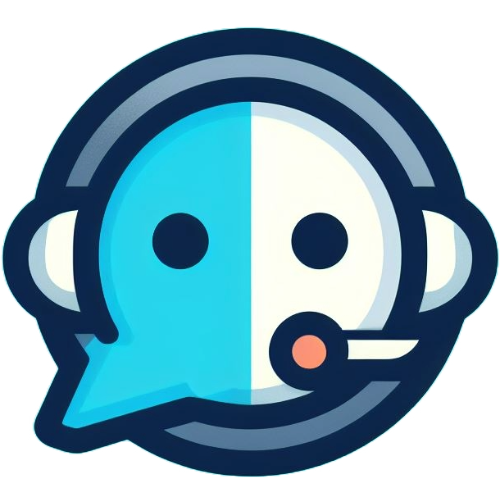

I need to give John some major KUDOS. I actually followed the link to his course and now, after like 10% in, I've learned at least 4 or 5 new things that are going to make my life so much easier. Thanks buddy for making these videos and adding a course. Ternary alone is going to save me so much time. A+++
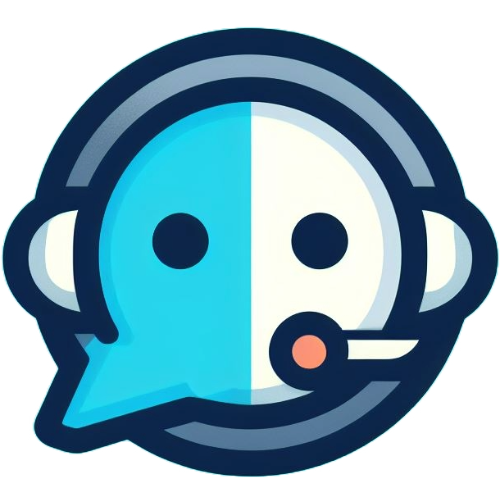

I think this was a missed opportunity to change all those calculator tests to one simple parameterized test so that people who are learning how to write unit test do correctly from the start. Your tests were good and basic but wouldn't make it past default sonar unfortunately
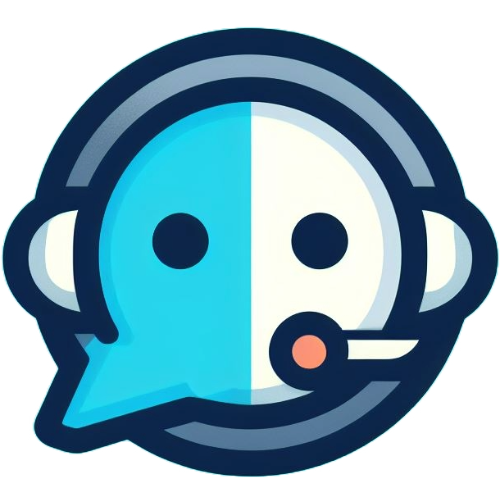